Note: All code examples on this site are provided for developer reference/guidance only and we cannot guarantee that they will always work as expected. Our support policy does not include assistance with modifying or debugging code from any code examples, and they may be changed or removed if we find they no longer work due to changes in our plugins.
This tutorial applies to users running both Job Manager Resumes and WC Paid Listings and lets you restrict access to the resumes portion of your site to users with an active job package only.
Configure Resume Access
The first step is to configure resumes for capability based access. Go to Resumes > Settings > Resume Visibility and for each permission enter ‘has_active_job_package’.
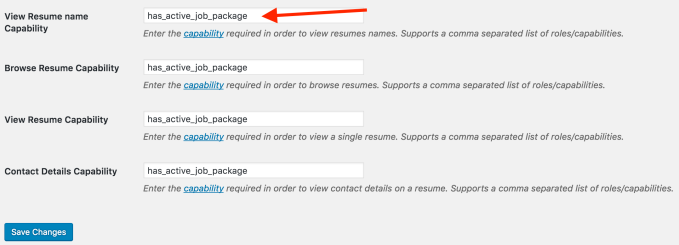
Add a snippet
The following code snippet can go either in a custom plugin, or your theme functions.php file.
<?php
// Hook into user_has_cap filter. This assumes you have setup resumes to require the capability 'has_active_job_package'
add_filter( 'user_has_cap', 'has_active_job_package_capability_check', 10, 3 );
/**
* has_active_job_package_capability_check()
*
* Filter on the current_user_can() function.
*
* @param array $allcaps All the capabilities of the user
* @param array $cap [0] Required capability
* @param array $args [0] Requested capability
* [1] User ID
* [2] Associated object ID
*/
function has_active_job_package_capability_check( $allcaps, $cap, $args ) {
// Only interested in has_active_job_package
if ( empty( $cap[0] ) || $cap[0] !== 'has_active_job_package' || ! function_exists( 'wc_paid_listings_get_user_packages' ) ) {
return $allcaps;
}
$user_id = $args[1];
$packages = wc_paid_listings_get_user_packages( $user_id, 'job_listing' );
// Has active package
if ( is_array( $packages ) && sizeof( $packages ) > 0 ) {
$allcaps[ $cap[0] ] = true;
}
return $allcaps;
}
What does it do? When WordPress checks if the user has the correct capability to view resumes, it checks the users packages. If they have a package, they are given the capability dynamically.
Modifying the snippet to also check for active job listings
If you also want to allow resume access if they have an active job listing i.e. one they have posted with their package, we can tweak this snippet to also check the listings they have posted.
<?php
// Hook into user_has_cap filter. This assumes you have setup resumes to require the capability 'has_active_job_package'
add_filter( 'user_has_cap', 'has_active_job_package_or_job_capability_check', 10, 3 );
/**
* has_active_job_package_or_job_capability_check()
*
* Filter on the current_user_can() function.
*
* @param array $allcaps All the capabilities of the user
* @param array $cap [0] Required capability
* @param array $args [0] Requested capability
* [1] User ID
* [2] Associated object ID
*/
function has_active_job_package_or_job_capability_check( $allcaps, $cap, $args ) {
// Only interested in has_active_job_package
if ( empty( $cap[0] ) || $cap[0] !== 'has_active_job_package' || ! function_exists( 'get_user_job_packages' ) ) {
return $allcaps;
}
$user_id = $args[1];
$packages = get_user_job_packages( $user_id );
// Has active package
if ( is_array( $packages ) && sizeof( $packages ) > 0 ) {
$allcaps[ $cap[0] ] = true;
}
// Has active job listing
if ( is_user_logged_in() ) {
$active_jobs = get_posts( array(
'post_type' => 'job_listing',
'post_status' => array( 'publish', 'pending' ),
'ignore_sticky_posts' => 1,
'posts_per_page' => 1,
'author' => get_current_user_id(),
'fields' => 'ids'
) );
if ( sizeof( $active_jobs ) > 0 ) {
$allcaps[ $cap[0] ] = true;
}
}
return $allcaps;
}
If they have an active job listing already posted, this will let them view resumes. When that job expires, this check will no longer return true.