Note: All code examples on this site are provided for developer reference/guidance only and we cannot guarantee that they will always work as expected. Our support policy does not include assistance with modifying or debugging code from any code examples, and they may be changed or removed if we find they no longer work due to changes in our plugins.
Note: Since WP Job Manager version 1.27.0, the job permalinks can be changed inside Settings -> Permalinks, under the Optional section.
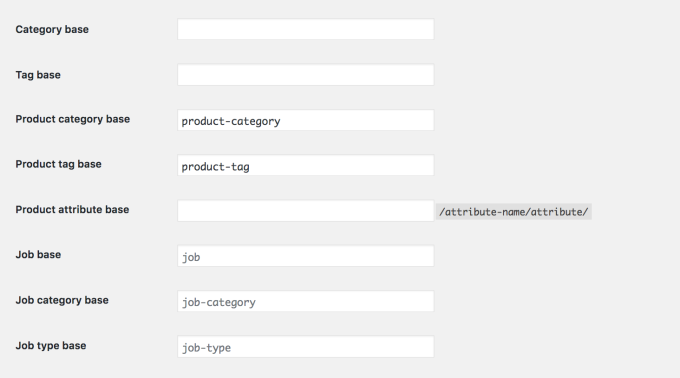
For the previous versions of WP Job Manager, please refer to the guide below. Thanks!
Job listings in WP Job Manager default to the permalink ‘jobs’. So, for example, a job may have the following URL: http://yoursite.com/jobs/job-listing-title
There are two methods of customising this;
- Using a localisation file and translating the string
- Using a filter
Important: After changing the slug, you need to resave your permalinks for it to come into effect. Go to your WordPress dashboard and find Settings > Permalinks. Hit save and you are done.
Changing the permalink base using filters
The filter method involves adding some custom code to your theme functions.php to filter the permalink. e.g. Change ‘jobs’ to ‘careers’:
<?php
function change_job_listing_slug( $args ) {
$args['rewrite']['slug'] = _x( 'careers', 'Job permalink - resave permalinks after changing this', 'job_manager' );
return $args;
}
add_filter( 'register_post_type_job_listing', 'change_job_listing_slug' );
Changing the permalink slug for new jobs
To make job permalinks unique, the slug has company name, location name, and job type (as a number) appended to it. e.g. job/acme-london-6-awesome-job
(Note: these are only added when the job is posted via the submit job form on the frontend).
You can customise this via filters added to your theme functions.php file:
<?php
// Remove company name
add_filter( 'submit_job_form_prefix_post_name_with_company', '__return_false' );
// Remove location
add_filter( 'submit_job_form_prefix_post_name_with_location', '__return_false' );
// Remove job type
add_filter( 'submit_job_form_prefix_post_name_with_job_type', '__return_false' );
Omit or comment out the parts you don’t want to remove from your job permalinks.
Example: Appending the Job ID to the permalink slug
This code will append the job ID to the permalink slug when saving a new job listing. e.g. e.g. job/acme-london-6-awesome-job-136
<?php
function custom_job_post_type_link( $post_id, $post ) {
// don't add the id if it's already part of the slug
$permalink = $post->post_name;
if ( strpos( $permalink, strval( $post_id ) ) ) {
return;
}
// unhook this function to prevent infinite looping
remove_action( 'save_post_job_listing', 'custom_job_post_type_link', 10, 2 );
// add the id to the slug
$permalink .= '-' . $post_id;
// update the post slug
wp_update_post( array(
'ID' => $post_id,
'post_name' => $permalink
));
// re-hook this function
add_action( 'save_post_job_listing', 'custom_job_post_type_link', 10, 2 );
}
add_action( 'save_post_job_listing', 'custom_job_post_type_link', 10, 2 );
Note: If you want to apply this to all existing job listings, you’ll need to bulk edit them and click update, to trigger the “save_post” action.
Example: Adding the Job ID to the base URL
This snippet would add the Job’s ID to the base url. E.g. /job/1234/job-title
<?php
function job_listing_post_type_link( $permalink, $post ) {
// Abort if post is not a job
if ( $post->post_type !== 'job_listing' )
return $permalink;
// Abort early if the placeholder rewrite tag isn't in the generated URL
if ( false === strpos( $permalink, '%' ) )
return $permalink;
$find = array(
'%post_id%'
);
$replace = array(
$post->ID
);
$replace = array_map( 'sanitize_title', $replace );
$permalink = str_replace( $find, $replace, $permalink );
return $permalink;
}
add_filter( 'post_type_link', 'job_listing_post_type_link', 10, 2 );
function change_job_listing_slug( $args ) {
$args['rewrite']['slug'] = 'job/%post_id%';
return $args;
}
add_filter( 'register_post_type_job_listing', 'change_job_listing_slug' );
Example: Adding the category to the base URL
This snippet would add the Job’s category name to the base url. E.g. /job/job-category/job-title
<?php
function job_listing_post_type_link( $permalink, $post ) {
// Abort if post is not a job
if ( $post->post_type !== 'job_listing' )
return $permalink;
// Abort early if the placeholder rewrite tag isn't in the generated URL
if ( false === strpos( $permalink, '%' ) )
return $permalink;
// Get the custom taxonomy terms in use by this post
$terms = wp_get_post_terms( $post->ID, 'job_listing_category', array( 'orderby' => 'parent', 'order' => 'ASC' ) );
if ( empty( $terms ) ) {
// If no terms are assigned to this post, use a string instead (can't leave the placeholder there)
$job_listing_category = _x( 'uncat', 'slug' );
} else {
// Replace the placeholder rewrite tag with the first term's slug
$first_term = array_shift( $terms );
$job_listing_category = $first_term->slug;
}
$find = array(
'%category%'
);
$replace = array(
$job_listing_category
);
$replace = array_map( 'sanitize_title', $replace );
$permalink = str_replace( $find, $replace, $permalink );
return $permalink;
}
add_filter( 'post_type_link', 'job_listing_post_type_link', 10, 2 );
function change_job_listing_slug( $args ) {
$args['rewrite']['slug'] = 'job/%category%';
return $args;
}
add_filter( 'register_post_type_job_listing', 'change_job_listing_slug' );
Example: Adding the category and region to the base URL
This snippet would add the category name and region name to the base url. E.g. /job/job-category/region/job-title
(Requires the Astoundify Predefined Regions plugin. Please note that this plugin has not been updated in 2 years, so there is no guarantee this snippet will work. Use at your own risk.)
<?php
function job_listing_post_type_link( $permalink, $post ) {
// Abort if post is not a job
if ( $post->post_type !== 'job_listing' ) {
return $permalink;
}
// Abort early if the placeholder rewrite tag isn't in the generated URL
if ( false === strpos( $permalink, '%' ) ) {
return $permalink;
}
// Get the custom taxonomy terms in use by this post
$categories = wp_get_post_terms( $post->ID, 'job_listing_category', array( 'orderby' => 'parent', 'order' => 'ASC' ) );
$regions = wp_get_post_terms( $post->ID, 'job_listing_region', array( 'orderby' => 'parent', 'order' => 'ASC' ) );
if ( empty( $categories ) ) {
// If no terms are assigned to this post, use a string instead (can't leave the placeholder there)
$job_listing_category = _x( 'uncategorized', 'slug' );
} else {
// Replace the placeholder rewrite tag with the first term's slug
$first_term = array_shift( $categories );
$job_listing_category = $first_term->slug;
}
if ( empty( $regions ) ) {
// If no terms are assigned to this post, use a string instead (can't leave the placeholder there)
$job_listing_region = _x( 'anywhere', 'slug' );
} else {
// Replace the placeholder rewrite tag with the first term's slug
$first_term = array_shift( $regions );
$job_listing_region = $first_term->slug;
}
$find = array(
'%category%',
'%region%'
);
$replace = array(
$job_listing_category,
$job_listing_region
);
$replace = array_map( 'sanitize_title', $replace );
$permalink = str_replace( $find, $replace, $permalink );
return $permalink;
}
add_filter( 'post_type_link', 'job_listing_post_type_link', 10, 2 );
function change_job_listing_slug( $args ) {
$args['rewrite']['slug'] = 'job/%category%/%region%';
return $args;
}
add_filter( 'register_post_type_job_listing', 'change_job_listing_slug' );
function add_region_endpoint_tag() {
add_rewrite_tag( '%region%', '([^/]*)' );
}
add_action( 'init', 'add_region_endpoint_tag' );
Change the job category and job type slugs
With the following snippet, you can change the slug for job category and job type. E.g. /job/industria/do-not-know/job-title
<?php
// Change the 'job-category' slug
add_filter( 'register_taxonomy_job_listing_category_args', 'change_job_listing_category_rewrite' );
function change_job_listing_category_rewrite( $options ) {
$options['rewrite'] = array(
'slug' => 'industria',
'with_front' => false,
'hierarchical' => false,
);
return $options;
}
// Change the 'job-type' slug
add_filter( 'register_taxonomy_job_listing_type_args', 'change_job_listing_type_rewrite' );
function change_job_listing_type_rewrite( $options ) {
$options['rewrite'] = array(
'slug' => 'do-not-know',
'with_front' => false,
'hierarchical' => false,
);
return $options;
}